Antarctic ice shelf basal melt¶
Results from Adumusulli et al. (2020), which can be found and downloaded here https://library.ucsd.edu/dc/object/bb0448974g
These load in the Adumusulli et al. (2020) data, stored as a zarr on the pangeo-glaciology Google bucket, using open_catalog
method.
[1]:
import numpy as np
import xarray as xr
import matplotlib.pyplot as plt
from intake import open_catalog
import hvplot.xarray
Initalize dataset¶
[2]:
cat = open_catalog('https://raw.githubusercontent.com/ldeo-glaciology/subshelf_meltrates/master/A2020_meltrates.yaml')
b = cat["A2020_meltrates"].to_dask()
b
[2]:
<xarray.Dataset> Dimensions: (x: 10941, y: 10229) Coordinates: * x (x) float64 -2.736e+06 -2.736e+06 ... 2.734e+06 2.734e+06 * y (y) float64 -2.374e+06 -2.374e+06 ... 2.74e+06 2.74e+06 Data variables: meltrates (y, x) float64 dask.array<chunksize=(320, 684), meta=np.ndarray>
xarray.Dataset
- x: 10941
- y: 10229
- x(x)float64-2.736e+06 -2.736e+06 ... 2.734e+06
array([-2736000., -2735500., -2735000., ..., 2733000., 2733500., 2734000.])
- y(y)float64-2.374e+06 -2.374e+06 ... 2.74e+06
array([-2374000., -2373500., -2373000., ..., 2739000., 2739500., 2740000.])
- meltrates(y, x)float64dask.array<chunksize=(320, 684), meta=np.ndarray>
Array Chunk Bytes 895.32 MB 1.75 MB Shape (10229, 10941) (320, 684) Count 513 Tasks 512 Chunks Type float64 numpy.ndarray
start a cluster to do some fast processing of this relatively small dataset¶
[3]:
from dask.distributed import Client
import dask_gateway
gateway = dask_gateway.Gateway()
cluster = gateway.new_cluster()
[4]:
cluster.scale(4)
client = Client(cluster)
cluster
to view the dask scheduler dashboard either click the link above, or copy the link in to the serach bar in the dask tab on the right, prefixing it with https://us-central1-b.gcp.pangeo.io/ - then you get access to all the tool and they can be docked in this window.¶
[5]:
bcoarse = b.meltrates.coarsen(y=10,x=10,boundary="trim").mean().persist()
[6]:
bcoarse.plot(vmin=-2,vmax=10)
[6]:
<matplotlib.collections.QuadMesh at 0x7f02f4bfd9d0>
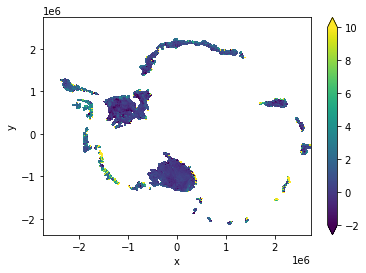
Antarctic ice shelf firn thickness¶
Results from Adumusulli et al. (2020), which can be found and downloaded here https://library.ucsd.edu/dc/object/bb0448974g
These load in the Adumusulli et al. (2020) data, stored as a zarr on the pangeo-glaciology Google bucket, using open_catalog
method.
[7]:
cat = open_catalog('https://raw.githubusercontent.com/ldeo-glaciology/subshelf_meltrates/master/A2020_deltaH.yaml')
dH = cat["A2020_deltaH"].to_dask()
dH
[7]:
<xarray.Dataset> Dimensions: (time: 107, x: 510, y: 436) Coordinates: * time (time) float64 1.992e+03 1.993e+03 ... 2.019e+03 2.019e+03 * x (x) float64 -2.36e+06 -2.35e+06 ... 2.72e+06 2.73e+06 * y (y) float64 -2.12e+06 -2.11e+06 ... 2.22e+06 2.23e+06 Data variables: h_alt (time, y, x) float64 dask.array<chunksize=(14, 109, 128), meta=np.ndarray> h_firn (time, y, x) float64 dask.array<chunksize=(14, 109, 128), meta=np.ndarray> smb_discharge (time, y, x) float64 dask.array<chunksize=(14, 109, 128), meta=np.ndarray> uncert_alt (time, y, x) float64 dask.array<chunksize=(14, 109, 128), meta=np.ndarray> uncert_firn (y, x) float64 dask.array<chunksize=(218, 255), meta=np.ndarray>
xarray.Dataset
- time: 107
- x: 510
- y: 436
- time(time)float641.992e+03 1.993e+03 ... 2.019e+03
array([1992.409589, 1992.660274, 1992.912329, 1993.161644, 1993.409589, 1993.660274, 1993.912329, 1994.161644, 1994.409589, 1994.660274, 1994.912329, 1995.161644, 1995.409589, 1995.660274, 1995.912276, 1996.162568, 1996.411202, 1996.661202, 1996.912621, 1997.161644, 1997.409589, 1997.660274, 1997.912329, 1998.161644, 1998.409589, 1998.660274, 1998.912329, 1999.161644, 1999.409589, 1999.660274, 1999.912276, 2000.162568, 2000.411202, 2000.661202, 2000.912621, 2001.161644, 2001.409589, 2001.660274, 2001.912329, 2002.161644, 2002.409589, 2002.660274, 2002.912329, 2003.161644, 2003.409589, 2003.660274, 2003.912276, 2004.162568, 2004.411202, 2004.661202, 2004.912621, 2005.161644, 2005.409589, 2005.660274, 2005.912329, 2006.161644, 2006.409589, 2006.660274, 2006.912329, 2007.161644, 2007.409589, 2007.660274, 2007.912276, 2008.162568, 2008.411202, 2008.661202, 2008.912621, 2009.161644, 2009.409589, 2009.660274, 2009.912329, 2010.161644, 2010.409589, 2010.660274, 2010.912329, 2011.161644, 2011.409589, 2011.660274, 2011.912329, 2012.161644, 2012.409589, 2012.660274, 2012.912329, 2013.161644, 2013.409589, 2013.660274, 2013.912329, 2014.161644, 2014.409589, 2014.660274, 2014.912329, 2015.161644, 2015.409589, 2015.660274, 2015.912329, 2016.161644, 2016.409589, 2016.660274, 2016.912329, 2017.161644, 2017.409589, 2017.660274, 2017.912329, 2018.161644, 2018.409589, 2018.660274, 2018.912329])
- x(x)float64-2.36e+06 -2.35e+06 ... 2.73e+06
array([-2360000., -2350000., -2340000., ..., 2710000., 2720000., 2730000.])
- y(y)float64-2.12e+06 -2.11e+06 ... 2.23e+06
array([-2120000., -2110000., -2100000., ..., 2210000., 2220000., 2230000.])
- h_alt(time, y, x)float64dask.array<chunksize=(14, 109, 128), meta=np.ndarray>
Array Chunk Bytes 190.34 MB 1.56 MB Shape (107, 436, 510) (14, 109, 128) Count 129 Tasks 128 Chunks Type float64 numpy.ndarray - h_firn(time, y, x)float64dask.array<chunksize=(14, 109, 128), meta=np.ndarray>
Array Chunk Bytes 190.34 MB 1.56 MB Shape (107, 436, 510) (14, 109, 128) Count 129 Tasks 128 Chunks Type float64 numpy.ndarray - smb_discharge(time, y, x)float64dask.array<chunksize=(14, 109, 128), meta=np.ndarray>
Array Chunk Bytes 190.34 MB 1.56 MB Shape (107, 436, 510) (14, 109, 128) Count 129 Tasks 128 Chunks Type float64 numpy.ndarray - uncert_alt(time, y, x)float64dask.array<chunksize=(14, 109, 128), meta=np.ndarray>
Array Chunk Bytes 190.34 MB 1.56 MB Shape (107, 436, 510) (14, 109, 128) Count 129 Tasks 128 Chunks Type float64 numpy.ndarray - uncert_firn(y, x)float64dask.array<chunksize=(218, 255), meta=np.ndarray>
Array Chunk Bytes 1.78 MB 444.72 kB Shape (436, 510) (218, 255) Count 5 Tasks 4 Chunks Type float64 numpy.ndarray
[8]:
dH.h_firn.hvplot.image('x', 'y',
groupby='time', rasterize=True, dynamic=True, width=800, height=600,
widget_type='scrubber', widget_location='bottom', cmap='RdBu_r')
[8]:
[ ]: